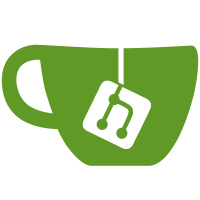
- Created global.css for styling with a Scandinavian industrial palette. - Added index.ejs as the main entry point for the application, featuring a form creation section and a list of existing forms. - Implemented main.js for dropdown and modal functionalities. - Introduced submissions.ejs to display submissions for each form with pagination and action buttons. - Ensured accessibility features such as skip links and ARIA attributes for better user experience.
71 lines
2.3 KiB
JavaScript
71 lines
2.3 KiB
JavaScript
// Dropdown functionality
|
|
function toggleDropdown(button) {
|
|
// Find the closest .dropdown and then the .dropdown-menu inside it
|
|
const dropdown = button.closest('.dropdown');
|
|
if (!dropdown) return;
|
|
const dropdownMenu = dropdown.querySelector('.dropdown-menu');
|
|
if (dropdownMenu) {
|
|
dropdownMenu.classList.toggle('show');
|
|
}
|
|
}
|
|
|
|
// Modal functionality
|
|
function showModal(modalId) {
|
|
document.getElementById(modalId).classList.add('show');
|
|
}
|
|
|
|
function hideModal(modalId) {
|
|
document.getElementById(modalId).classList.remove('show');
|
|
}
|
|
|
|
// Initialize all event listeners
|
|
document.addEventListener('DOMContentLoaded', function () {
|
|
// Handle dropdown toggles
|
|
document.querySelectorAll('[data-action="toggle-dropdown"]').forEach(button => {
|
|
button.addEventListener('click', function () {
|
|
toggleDropdown(this);
|
|
});
|
|
});
|
|
|
|
// Handle modal show buttons
|
|
document.querySelectorAll('[data-action="show-modal"]').forEach(button => {
|
|
button.addEventListener('click', function () {
|
|
const modalId = this.dataset.modal;
|
|
showModal(modalId);
|
|
});
|
|
});
|
|
|
|
// Handle modal hide buttons
|
|
document.querySelectorAll('[data-action="hide-modal"]').forEach(button => {
|
|
button.addEventListener('click', function () {
|
|
const modalId = this.dataset.modal;
|
|
hideModal(modalId);
|
|
});
|
|
});
|
|
|
|
// Handle test notification buttons
|
|
document.querySelectorAll('[data-action="test-notification"]').forEach(button => {
|
|
button.addEventListener('click', function () {
|
|
const formId = this.dataset.formId;
|
|
// Implement test notification functionality here
|
|
console.log('Testing notification for form:', formId);
|
|
});
|
|
});
|
|
|
|
// Close dropdowns when clicking outside
|
|
document.addEventListener('click', function (event) {
|
|
// Only close if click is outside any .dropdown
|
|
if (!event.target.closest('.dropdown')) {
|
|
document.querySelectorAll('.dropdown-menu.show').forEach(menu => {
|
|
menu.classList.remove('show');
|
|
});
|
|
}
|
|
});
|
|
|
|
// Close modals when clicking outside
|
|
document.addEventListener('click', function (event) {
|
|
if (event.target.classList.contains('modal')) {
|
|
event.target.classList.remove('show');
|
|
}
|
|
});
|
|
}); |